Create a function in JavaScript
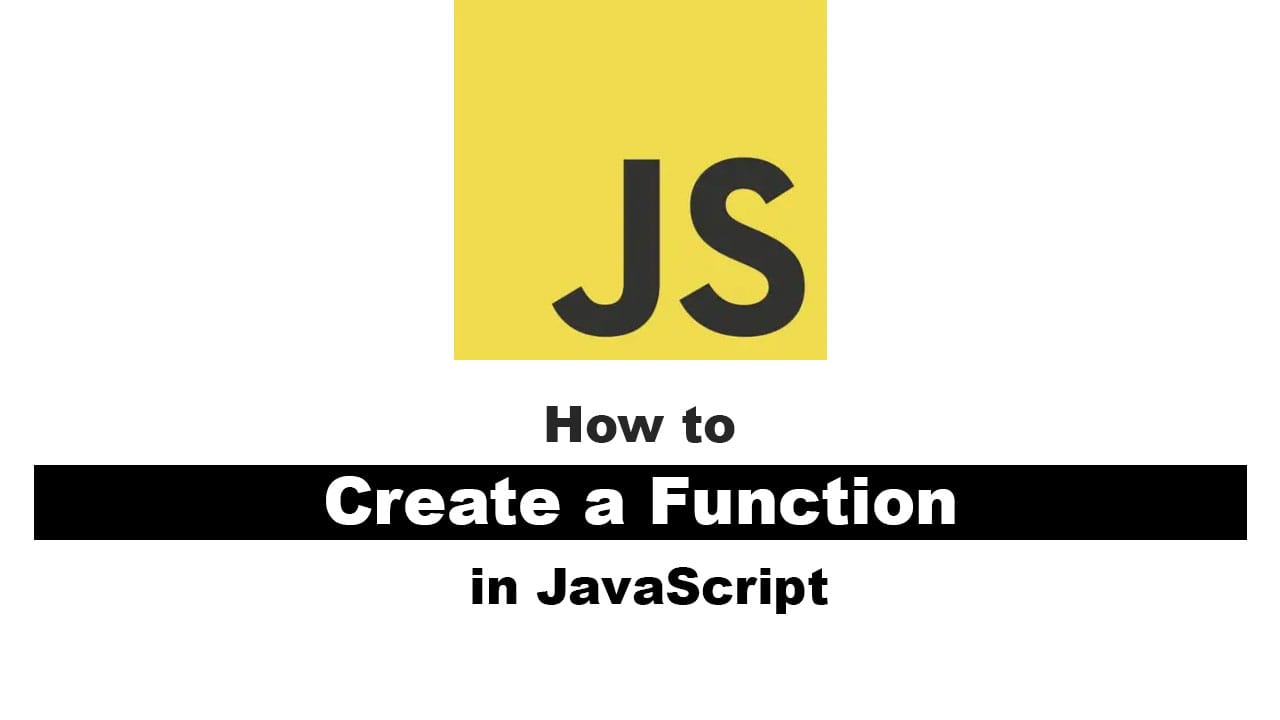
In JavaScript, there are three primary ways to create a function: Function Declaration, Function Expression, and Arrow Function.
- Function Declaration:
Example Code:
function sayHello() {
console.log("Hello, World!");
}
It is hosted and you can call a function before it’s defined in the code, and it will still work.
Characteristics: One of the major features of function declarations are hoisted to the top of their scope. Which mean, you can call a function before it’s defined in the code, and it will still work.
- Function Expression:
Example Code:
const add = function(a, b) {
return a + b;
};
console.log(add(5, 3)); // Outputs: 8
It is NOT hosted and you can NOT call a function before it’s defined.
Characteristics: Function expressions are NOT hoisted to the top of their scope. Which mean, you can NOT call a function before it’s defined in the code.
Function expressions are commonly used as Callbacks arguments to other functions or in situations where you need to define a function dynamically.
- Arrow Function (ES6+):
More concise syntax for writing functions, especially for small, simple functions. Arrow functions are always anonymous.
Example Code:
const multiply = (a, b) => a * b;
console.log(multiply(4, 5)); // Outputs: 20
Arrow functions are always anonymous.
Characteristics: Arrow functions don’t have their own this
context.
Example of Anonymous Function:
const multiply = function(a, b) {
return a * b;
};
console.log(multiply(4, 5)); // Outputs: 20
The anonymous function has no name
In this case, the anonymous function has no name, but it is stored in the multiply
variable, so you can call it through the variable.
Summary:
Feature | Function Declaration | Function Expression | Arrow Function |
---|---|---|---|
Hoisting | Yes | No | No |
Anonymous | No | Can be | Always anonymous |
this binding | Dynamic | Dynamic | Lexical |
Arguments object | Yes | Yes | No (use rest parameters) |
Use cases | General purpose, hoisted | Dynamic, callbacks | Concise functions, this context |
Do you enjoy this blog post?