How to dynamically add or remove object properties in JavaScript
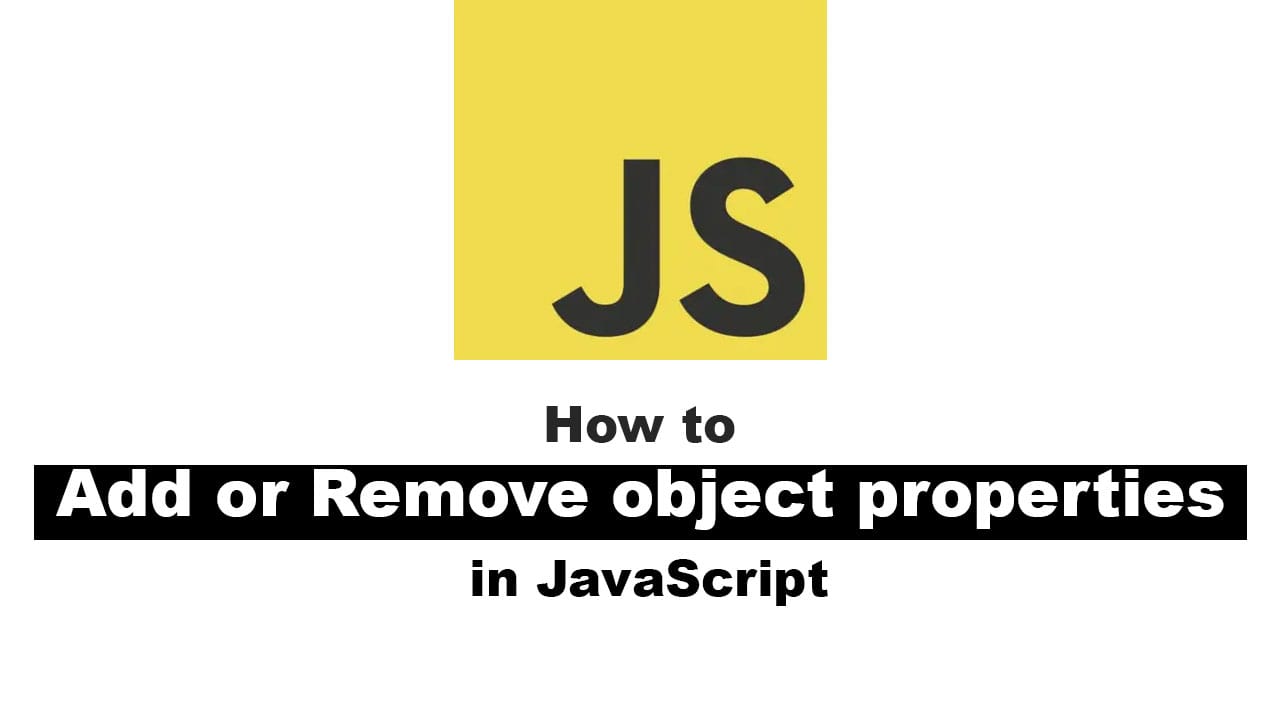
To add a new property:
There are four ways to add a new property to an object in JavaScript:
- Using Dot notation:
const obj = {name: "daviblog", age: 29 };
obj.job = "Programmer";
// obj = {name: "daviblog", age: 29, job: "Programmer"}
use Dot notation to add a new property
- Using Bracket notation:
const obj = {name: "daviblog", age: 29 };
obj["job"] = "Programmer";
// obj = {name: "daviblog", age: 29, job: "Programmer"}
use Bracket notation to add a new property
- Using Object.assign():
const obj = {name: "daviblog", age: 29 };
const newObj = Object.assign({},obj,{job:"Programmer"});
console.log(obj);
// Output: {name: "daviblog", age: 29, job: "Programmer"}
use Object.assign() to add a new property
- Using Spread operation:
const obj = {name: "daviblog", age: 29 };
const newObj = {...obj, job:"Programmer"};
console.log(obj);
// Output: {name: "daviblog", age: 29, job: "Programmer"}
using Spread operation to add a new property
To delete a property:
You can delete a property by using Dot notation and Bracket notation:
- Using Dot notation:
const obj = {name: "daviblog", age: 29, job: "Programmer" };
delete obj.job;
console.log(obj);
// Output: {name: "daviblog", age: 29}
use Dot notation to delete a property
- Using Bracket notation:
const obj = {name: "daviblog", age: 29, job: "Programmer" };
delete obj["job"];
console.log(obj);
// Output: {name: "daviblog", age: 29}
use Bracket notation to add a property
To Add or Deleting Multiple Properties Dynamically:
To Add multiple Properties:
- Using Object.assign():
const obj = {name: "daviblog", age: 29, job: "Programmer" };
const newProps = {title: "senior", experience: "5 years" };
// Adding multiple properties using Object.assign() to new Object
const newObj = Object.assign({}, obj, newProps);
console.log(newObj);
// Output: { name: "daviblog", age: 29, job: "Programmer", title: "senior", experience: "5 years" }
- Using Spread Operation:
const obj = {name: "daviblog", age: 29, job: "Programmer" };
const newProps = {title: "senior", experience: "5 years" };
const newObj = {...obj, ...newProps};
console.log(newObj);
// Output: { name: "daviblog", age: 29, job: "Programmer", title: "senior", experience: "5 years" }
To Delete multiple Properties:
const obj = { name: "daviblog", age: 29, job: "Programmer", title: "senior", experience: "5 years" };
const propsToDelete = ["name", "title"];
propsToDelete.forEach(prop => {
delete obj[prop];
});
console.log(obj);
// Output: { age: 29, job: "Programmer", experience: "5 years" }