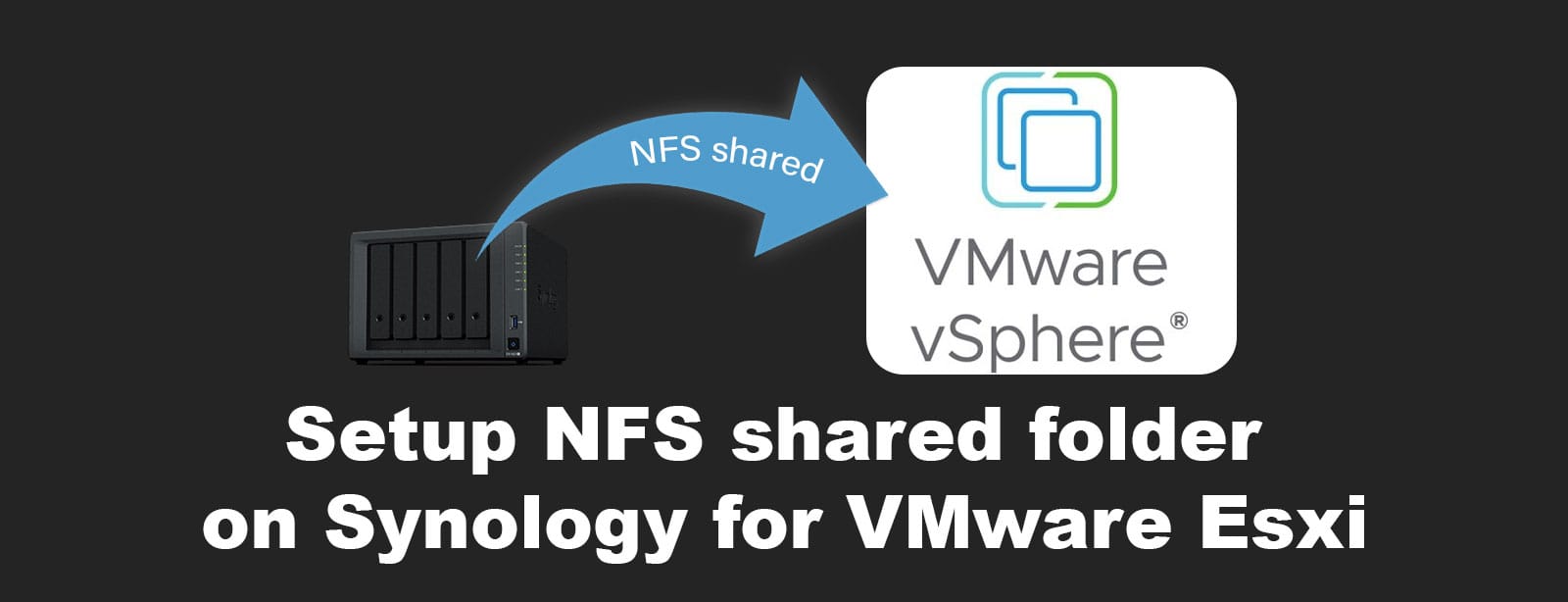
Synology
Setup NFS shared folder on Synology for VMware Esxi
Network File System (NFS) is a powerful way to create shared storage for VMware ESXi hosts. If you're looking to set up an NFS datastore on your Synology NAS and integrate it with VMware ESXi, this guide walks you through the process step by step. Let’s get